SwiftUI FileManager Access: A Comprehensive Guide
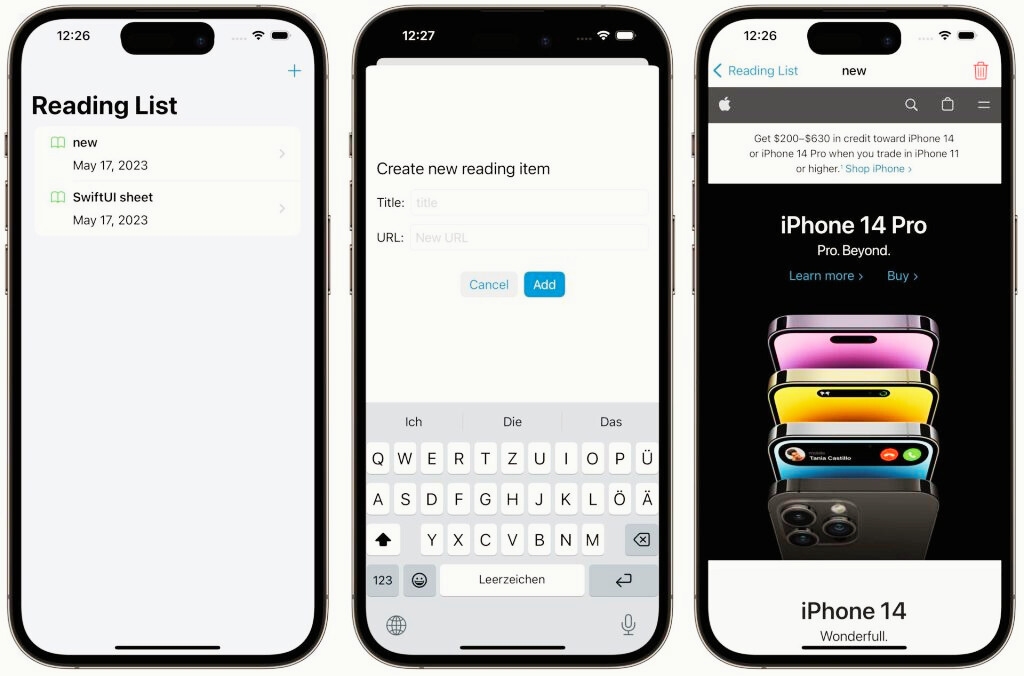
Introduction
Managing files and directories is a common requirement in iOS and macOS applications. SwiftUI itself does not provide direct file management APIs, but you can use FileManager, a Foundation framework class, to perform operations such as creating, reading, writing, listing, and deleting files. In this guide, we will explore how to use FileManager in a SwiftUI project.
1. Understanding the File System in iOS & macOS
Before diving into FileManager, it’s essential to understand where an app can store files:
- Documents Directory: Used for storing user-generated files (persistent across app launches).
- Caches Directory: Stores temporary files that can be deleted by the system when storage is low.
- Temporary Directory: Holds files that do not need to persist beyond the app session.
To get the path of these directories, use:
func getDocumentsDirectory() -> URL {
FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first!
}
func getCachesDirectory() -> URL {
FileManager.default.urls(for: .cachesDirectory, in: .userDomainMask).first!
}
func getTemporaryDirectory() -> URL {
FileManager.default.temporaryDirectory
}
2. Writing Data to a File
To write text data to a file in the Documents directory:
func saveFile(filename: String, content: String) {
let fileURL = getDocumentsDirectory().appendingPathComponent(filename)
do {
try content.write(to: fileURL, atomically: true, encoding: .utf8)
print(“File saved successfully!”)
} catch {
print(“Error saving file: \(error)”)
}
}
Example Usage:
saveFile(filename: “example.txt”, content: “Hello, SwiftUI!”)
3. Reading Data from a File
To read text from a file:
func readFile(filename: String) -> String? {
let fileURL = getDocumentsDirectory().appendingPathComponent(filename)
return try? String(contentsOf: fileURL, encoding: .utf8)
}
Example Usage:
if let content = readFile(filename: “example.txt”) {
print(“File Content: \(content)”)
}
4. Deleting a File
To delete a file from the Documents directory:
func deleteFile(filename: String) {
let fileURL = getDocumentsDirectory().appendingPathComponent(filename)
do {
try FileManager.default.removeItem(at: fileURL)
print(“File deleted successfully!”)
} catch {
print(“Error deleting file: \(error)”)
}
}
Example Usage:
deleteFile(filename: “example.txt”)
5. Listing Files in a Directory
To get a list of all files in the Documents directory:
func listFiles() -> [String] {
let documentsURL = getDocumentsDirectory()
let fileManager = FileManager.default
do {
let files = try fileManager.contentsOfDirectory(atPath: documentsURL.path)
return files
} catch {
print(“Error listing files: \(error)”)
return []
}
}
Example Usage:
let files = listFiles()
print(“Files: \(files)”)
6. Checking If a File Exists
Before reading or deleting a file, it’s good practice to check if it exists:
func fileExists(filename: String) -> Bool {
let fileURL = getDocumentsDirectory().appendingPathComponent(filename)
return FileManager.default.fileExists(atPath: fileURL.path)
}
Example Usage:
if fileExists(filename: “example.txt”) {
print(“File exists!”)
} else {
print(“File does not exist.”)
}
7. Secure File Access & Sandboxing
Since iOS apps run in a sandbox, they cannot access files outside their designated directories without user permission. To allow users to choose external files, use:
import UIKit
import UniformTypeIdentifiers
struct DocumentPicker: UIViewControllerRepresentable {
func makeCoordinator() -> Coordinator {
return Coordinator()
}
func makeUIViewController(context: Context) -> UIDocumentPickerViewController {
let picker = UIDocumentPickerViewController(forOpeningContentTypes: [UTType.text])
picker.delegate = context.coordinator
return picker
}
func updateUIViewController(_ uiViewController: UIDocumentPickerViewController, context: Context) {}
class Coordinator: NSObject, UIDocumentPickerDelegate {
func documentPicker(_ controller: UIDocumentPickerViewController, didPickDocumentsAt urls: [URL]) {
if let selectedFile = urls.first {
print(“Picked file: \(selectedFile)”)
}
}
}
}
Use DocumentPicker() inside a SwiftUI view to present the file picker UI.
8. Implementing a Simple SwiftUI File Manager UI
To integrate file management in SwiftUI, create a basic interface:
import SwiftUI
struct FileManagerView: View {
@State private var files: [String] = []
var body: some View {
VStack {
Button(“Save File”) {
saveFile(filename: “test.txt”, content: “SwiftUI FileManager Example”)
files = listFiles()
}
Button(“Load Files”) {
files = listFiles()
}
List(files, id: \ .self) { file in
Text(file)
}
}
.onAppear {
files = listFiles()
}
}
}
struct FileManagerView_Previews: PreviewProvider {
static var previews: some View {
FileManagerView()
}
}
Conclusion
Using FileManager in SwiftUI allows you to handle files efficiently, including saving, reading, deleting, and listing files in the app’s directories. By understanding sandboxing rules and utilizing UIDocumentPickerViewController where necessary, you can enhance your app’s file management capabilities securely.
Try incorporating these techniques into your SwiftUI projects to improve user file handling! 🚀